AJAX (Asyncronous JavaScript and XML)
|
AJAX (Asynchronous JavaScript and XML) is a web development technique intended to make web pages more responsive by exchanging small amounts of data with the web server without having to do a full post-back (reloading of the page). This technology is used to make a single web page more usable, responsive, and interactive. [4] |
|
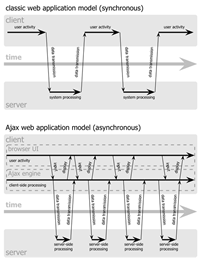
Figure 1: AJAX Web application model vs. Classic Web Application Model
|
|
|
The term AJAX was first discovered in 2005, but much of the technologies involved started a decade earlier by Microsoft’s initiatives in developing Remote Scripting (MSRS). The first use of the term in public was used by Jesse James Garrett in February 2005, who thought of the term to represent the suite of technologies he was proposing to a client [4]. AJAX was made popular by Google Maps, which uses AJAX to load pieces of user-requested maps, making their interfaces smoother. |
|
AJAX is not a technology, but rather it is a technique that calls upon a group of technologies [4]. AJAX uses a combination of:
- Client-side scripting language (JavaScript).
- HTML for markup.
- Optionally, CSS for styling information within HTML.
- An object called XMLHttpRequest is used to exchange data with a web server.
- XML is most commonly the format used to exchange data, but any custom format can be used.
|
|
Below is a simple example [3] of the AJAX style using HTML, JavaScript, and the XMLHttpRequest object.
Code
<html>
<body>
<script type="text/javascript">
function ajaxFunction()
{
var xmlHttp;
try
{
// Firefox, Opera 8.0+, Safari
xmlHttp=new XMLHttpRequest();
}
catch (e)
{
// Internet Explorer
try
{
xmlHttp=new ActiveXObject("Msxml2.XMLHTTP");
}
catch (e)
{
try
{
xmlHttp=new ActiveXObject("Microsoft.XMLHTTP");
}
catch (e)
{
alert("Your browser does not support AJAX!");
return false;
}
}
}
xmlHttp.onreadystatechange=function()
{
if(xmlHttp.readyState==4) // If the data exchange is complete
{
//Do something
}
}
xmlHttp.open("GET","time.asp",true);
xmlHttp.send(null);
}
</script>
<form name="myForm">
Name: <input type="text"
onkeydown="ajaxFunction();" name="username" />
Time: <input type="text" name="time" />
</form>
</body>
</html> |
Example Explained
The JavaScript function ajaxFunction() is called when the ‘onkeydown’ event is triggered on the “username” texbox within the HTML form “myForm”. Since ajaxFunction() is a client-side script, a post-back to the server is not requested at the time of calling. The event is triggered and the ajaxFunction() creates a new XMLHttpRequest object. Note that all browsers except Microsoft Internet Explorer use XMLHttpRequest. Internet Explorer uses an object called ActiveXObject instead, so we place a check in the ajaxFunction() for which browser is being used. When the object is created, the “onreadystatechanged” property holds a function that processes the response from the server. The open() method is then called with three arguments:
- The method to use when sending the request (GET or POST). Read on HTML Forms for explanations of these features.
- The URL of the server-side script to execute at the server. In this example, a time.asp page is implied and carries a simple asp function to return the server’s current time.
- Specifies whether or not the request should be handled asynchronously. In AJAX’s case, this should be set to true.
The send() method is then called which allows the client-side script (ajaxFunction()) to communicate with the server without having the page request a post-back and reload.
|
|
|
User Experience
AJAX greatly improves the user experience. No longer do web pages need to reload every time data is required from the server and therefore server-side operations no longer appear “heavy” [2] . The users can also interact with other parts of the web page while requests for data from the server are being processed asynchronously.
Minimal Data Transfer
By not performing a full postback and sending all page data, bandwidth usage is greatly reduced. Also, limited processing on the server is done with the fact that only necessary data is sent to the server. Not all form elements are processed, nor is the entire viewstate, images, etc. [1] |
|
Complex JavaScript
Building AJAX-enabled applications requires great knowledge of JavaScript. Also, the lack of debugging tools available for client-side scripting makes development more complicated. [1]
Breaks the Page Paradigm
AJAX requires a different view of a website. AJAX turns web pages, which are normally dependent upon state management, into more like desktop applications. The back button and bookmarking no longer work as expected because the traditional page lifecycle has been replaced with an AJAX-enabled page. Developers need to accommodate for this change to allow for state-dependant features to work properly [2]. |
|
- Helium: Advantages and Disadvantages of AJAX. http://www.helium.com/tm/49433/advantages-enabled-applications-classic
- MSDN Forums: Advantages of Using AJAX?. http://forums.microsoft.com/MSDN/ShowPost.aspx?PostID=1338514&SiteID=1
- W3Schools: AJAX Example. http://www.w3schools.com/ajax/ajax_example.asp
- Wikipedia, the free encyclopedia. http://en.wikipedia.org/wiki/AJAX
|
|
- Web Server Security. http://www.cas.mcmaster.ca/~wmfarmer/SE-4C03-07/wiki/sinnark/web.html
|
|
- AJAX: The Official Microsoft ASP.NET AJAX Site. http://ajax.asp.net/
- Asynchronous JavaScript and XML with the Java Platform. http://java.sun.com/developer/technicalArticles/J2EE/AJAX/
- W3Schools: AJAX Tutorial. http://www.w3schools.com/ajax/default.asp
- Google Maps (Example of AJAX in Action). http://maps.google.com/
- JavaScript (TM) - The Definitive JavaScript Resource. http://www.javascript.com/
|